Kolmogorov Complexity
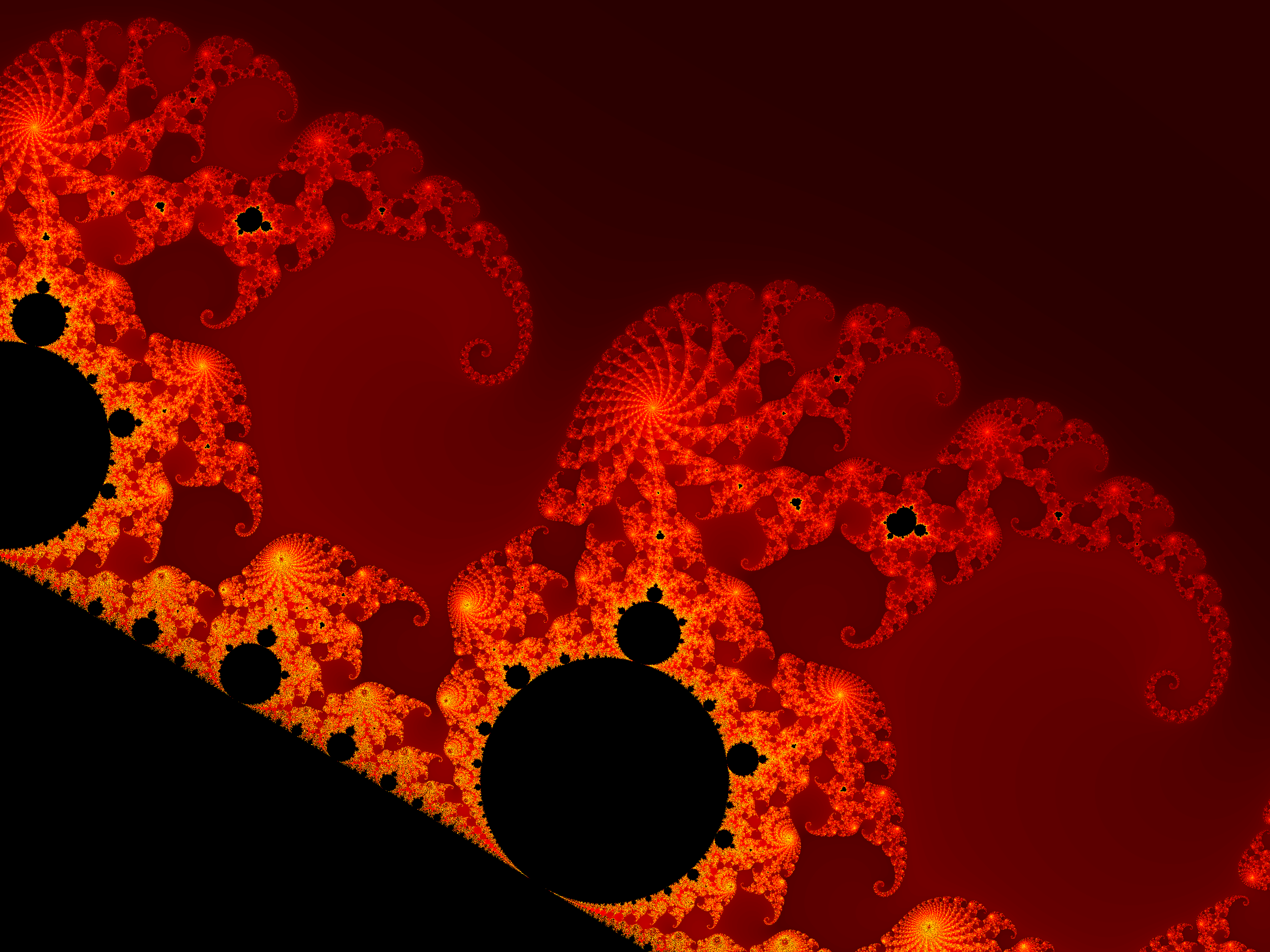
What Is Kolmogorov Complexity?
Think of Kolmogorov Complexity as a way to measure the complexity of a string (a sequence of characters) by looking at the length of the shortest possible program that can produce that string. Imagine you have a super-efficient computer program that generates text. Kolmogorov Complexity is about finding the tiniest program that can spit out the exact text you have.
Why It Matters
-
Kolmogorov Complexity is a big deal in algorithmic information theory, which is all about studying the information content of objects using computers. It helps us understand and measure randomness, information, and complexity in a very precise way.
-
In the world of data compression, Kolmogorov Complexity tells us the theoretical limit of how much we can compress a piece of data. If you know the complexity of a string, you know the smallest size you can squash it down to without losing any information.
-
A string with high Kolmogorov Complexity is considered random because there’s no shorter way to describe it than just writing it out. This matches our everyday idea of randomness, where random things don’t have obvious patterns and can’t be easily compressed.
-
Kolmogorov Complexity and entropy both measure information, but they do it in different ways. Entropy deals with the average uncertainty in a set of possible outcomes, like predicting the next letter in a text. Kolmogorov Complexity, on the other hand, is about the exact information content of a specific string.
-
One big challenge with Kolmogorov Complexity is that it’s not computable. We can’t always figure out the exact complexity of a string with an algorithm. However, there are related concepts and approximate methods that can be useful in practical situations.
// Pseudocode to illustrate the concept of Kolmogorov Complexity
function UniversalTuringMachine(program):
// Simulate the execution of the program to produce an output string
output = executeProgram(program)
return output
function EstimateKolmogorovComplexity(targetString):
// Initialize variables
shortestProgram = null
shortestLength = infinity
// Generate a set of potential programs
for program in generatePrograms():
// Simulate running the program on the universal Turing machine
output = UniversalTuringMachine(program)
// Check if the program produces the target string
if output == targetString:
// Check if this program is the shortest one found so far
if length(program) < shortestLength:
shortestProgram = program
shortestLength = length(program)
// Return the length of the shortest program found
return shortestLength
function generatePrograms():
// This function should generate all possible programs in increasing order of length
// For simplicity, we'll assume it generates a finite set of programs
programs = ["program1", "program2", "program3", ...]
return programs